시각화¶
Matplotlib¶
matplotlib는 Figure와 Axes 객체로 구성됩니다. Figure
는 그림이 보여지는 창 window을 의미하고 Axes
은 그 창 안에 보여지는 그림을 의미합니다. Axes
은 반드시 하나의 Figure
(창)에만 포함되어야 합니다. 하나의 Figure
는 여러 개의 Axes
을 포함할 수 있습니다.
빠르게 Figure
와 Axes
를 얻는 방법은 matplotlib.pyplot
을 이용하는 겁니다.
In [1]: import matplotlib.pyplot as plt
...:
...: fig, ax = plt.subplots()
...:
또는 plt.figure()
를 이용해서 Figure
객체를 얻을 수 있습니다.
In [2]: fig = plt.figure()
...:
plt.figure()
를 호출할 때마다 새로운 Figure
객체가 하나씩 생성됩니다. 만들어진 Figure
객체에 접근하기 위해서는 plt.figure(1)
과 같이 만들어진 순번을 입력하면 됩니다. plt.figure('one')
과 같이 Figure
를 만들 때 이름을 설정하면 나중에 접근할 때 만들어진 순서 번호로 접근할 수도 있고 지정된 이름으로도 접근할 수 있습니다.
만들어진 Figure
에 그림을 그리고 싶으면 Axes
객체를 만들어야 합니다. Axes
객체는 대부분의 플롯 함수들 plot
, scatter
, step
, bar
, stem
, pie
, boxplot
, hist
, countour
등을 가지고 있습니다. Axes
객체는 fig.gca()
메소드를 이용해서 새로운 Axes
을 만들거나 기존의 Axes
을 접근할 수 있습니다.
In [3]: ax1 = fig.gca()
...:
Font¶
폰트는 font_manager를 이용합니다.
시스템 폰트를 찾는 방법은 matplotlib.font_manager를 이용합니다.
In [4]: from matplotlib import font_manager
...:
...: fonts = font_manager.findSystemFonts()
...: fonts[:5]
...:
Out[4]:
['C:\\WINDOWS\\Fonts\\HATTEN.TTF',
'C:\\Windows\\Fonts\\trebucit.ttf',
'C:\\WINDOWS\\Fonts\\VINERITC.TTF',
'C:\\WINDOWS\\Fonts\\LeelUIsl.ttf',
'C:\\Windows\\Fonts\\ROCCB___.TTF']
malgun
폰트가 있는지 확인해 봅니다.
In [5]: [font for font in fonts if 'malgun' in font]
...:
Out[5]:
['C:\\Windows\\Fonts\\malgun.ttf',
'C:\\WINDOWS\\Fonts\\malgunbd.ttf',
'C:\\WINDOWS\\Fonts\\malgunsl.ttf',
'C:\\Windows\\Fonts\\malgunbd.ttf',
'C:\\Windows\\Fonts\\malgunsl.ttf',
'C:\\WINDOWS\\Fonts\\malgun.ttf']
폰트를 설정합니다. rc
는 리소스 설정을 하는 함수입니다.
In [6]: from matplotlib import rc
...:
...: font_name = font_manager.FontProperties(fname='C:\\Windows\\Fonts\\malgun.ttf').get_name()
...: print(font_name)
...: rc('font', family=font_name)
...:
Malgun Gothic
한글이 출력되는 것을 확인할 수 있습니다.
In [7]: import matplotlib.pyplot as plt
...:
...: fig, ax = plt.subplots()
...:
...: ax.plot([1, 2, 3], [3, 2, 1], label='한글 테스트')
...: ax.legend()
...:
Out[7]: <matplotlib.legend.Legend at 0x2825ef8e580>
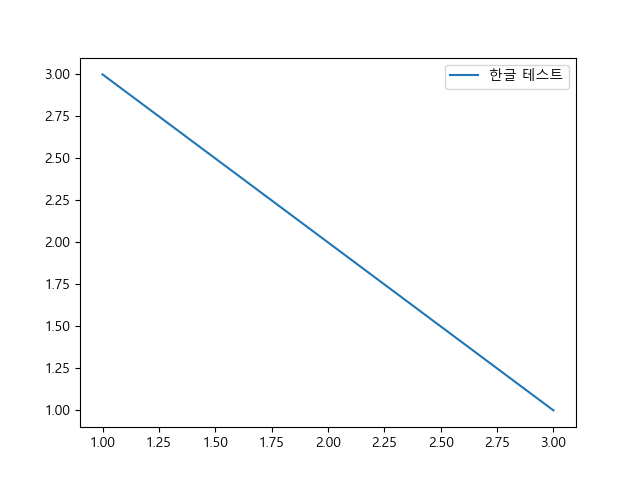